Introduction To Micro Frontend. Part I
12 May 2021
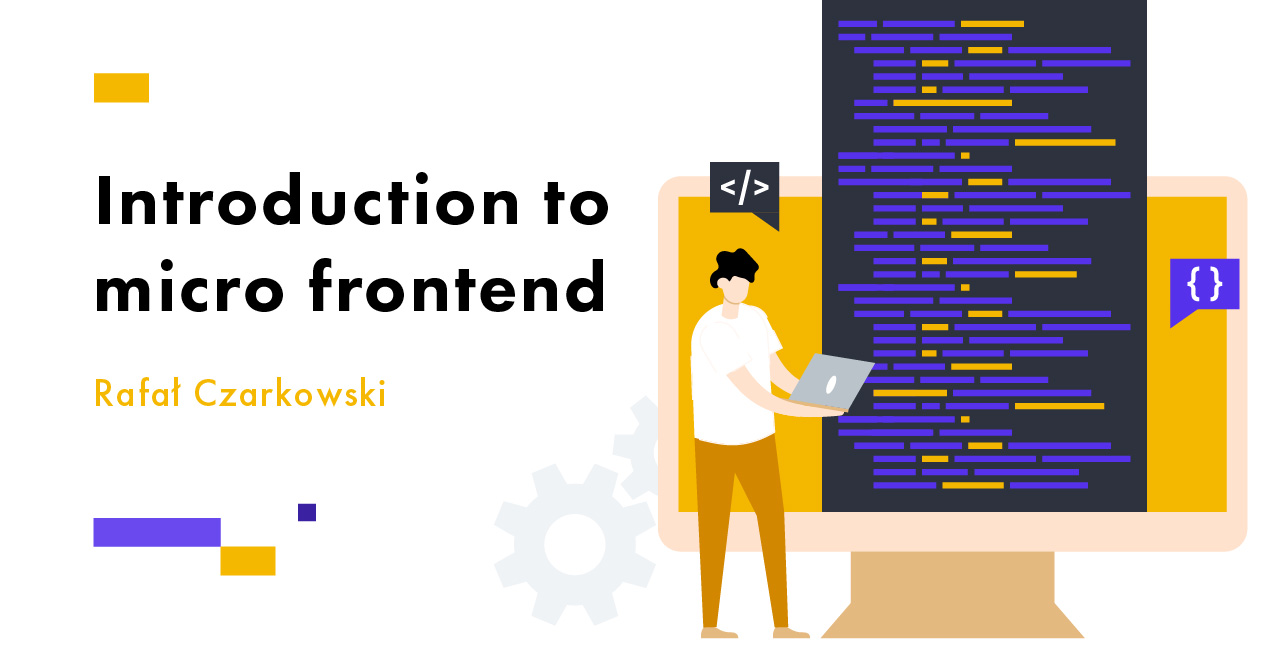
1. Introduction, a few words about micro frontend architecture, solution architecture
Micro frontends are small web applications that are broken down into components or functions that work together to provide a larger application.
If your project is small enough and supported by one team, the better solution will be to choose a monolithic SPA architecture and use one favorite framework such as Angular, React, Vue, Svelte etc. to build the presentation layer.
If the project starts to grow or several teams will work on it, it is definitely worth taking an interest in the micro frontend solution, which will facilitate the work and coordination of micro-projects by individual teams. Each team, according to the project specification, can use a different preferred framework.
Teams can have their own functions and components, work in their own code base and use their own dependencies in the project, release versions independently, constantly deliver small, incremental updates.
When using a micro frontend solution, we must also remember in what contexts they are most beneficial.
Martin Fowler defined Micro-Frontend Architecture as “an architectural style in which independently delivered frontend applications are assembled into a larger whole.”
Also, remember that everything has its advantages and disadvantages.
Choose solutions to the needs of the project carefully.
2. Theory in application, what problems it solves, micro frontend vs monolith SPA
Let’s look at the simplified diagram below, which will tell us a lot about the architecture of the solution and the approach to micro-applications and these components.
Source: https://micro-frontends.org
On the left, we can see the standard approach using a monolith as our application which includes a presentation layer, a data access layer, a logic layer, etc.
This is a standard approach used in small applications with the coordination of one team called Monolith.
In the middle part we see the front and back end layers.
The project has been divided into two teams that communicate with each other, but their responsibility has been divided into the area they deal with.
On the right, we have an approach to the project as microservices.
We have a dedicated part of the frontend and shared access to dedicated services.
Below in the diagram, we see the approach to the project as a micro frontend.
Source: https://micro-frontends.org
Each area is separated into a team that deals with a separate functionality, e.g. data searching or providing information from the newsletter.
The approach is great when several teams are working on a project.
Responsibility comes down to the preparation of the appropriate component / functionality that will be used in the project.
An additional advantage is the creation by teams of independent separate micro-projects that have their own dependencies and can be implemented using other javascript frameworks, e.g. angular, react, etc.
Source codes are separated into independent projects, which makes it easier to upgrade the version, update sources or replace old legacy components with new ones without interfering with the operation of our application.
So what are the advantages of using a micro frontend?
The most important ones that appear and are appreciated by the mass of programmers are:
– easy-to-maintain code
Using the micro frontend approach, the entire main application is divided into areas that are created by independent development teams that pursue a specific business goal.
These separated areas are easier to manage, update and change.
– independent placement
The ability to implement a small set of functions without being dependent on other modules significantly reduces the scope and complexity of the implementation, even if another micro-application has damaged components or functions.
– independent teams
Independent teams working on a specific solution and focusing on a designated area for which they are responsible.
Several teams from different time zones can work on the application, thanks to the fact that the application is separated into small projects, communication with teams is reduced to a minimum.
– scalable team of programmers
When the application receives new functionalities, we can flexibly implement new teams that will deal with individual functions and components without affecting the work of other teams.
Choosing to implement a project as micro fronted also has several disadvantages such as
– duplicate code
When creating micro applications, teams work using their dependencies in projects, which often results in a large increase in the libraries used and their duplication.
– state management
Each micro-application manages its own state.
In the traditional approach, the state is shared.
If your team needs to use shared state, you should take care of techniques that will help you encode URLs.
– communication between micro applications
Micro frontends are based on the concept of independent applications.
If we have a situation that our micro-application needs to transmit notifications to another micro-application should use the appropriate events, which may cause problems with synchronization.
– UI/UX consistency
If several teams scattered around the world with their own timezone are working on a project, problematic situations may arise, such as making change decisions in our main application. One team will want to make contextual changes to the application, which must be approved by other teams.
– Charging time
This can become an issue where we hate the separate micro project approach.
Each micro project has its own dependencies that the user must download for the application to work properly, and this extends the loading time of the entire application as an application
Of course, there are more advantages and disadvantages, I wanted to highlight only the most important ones in my opinion.
Here is a list of the most famous frameworks that already exist and that will make it easier when we are working on applications using the micro frontend approach.
- Webpack Module Federation
- Single SPA
- SystemJS
- Piral
- Open Components \ Web components
- Qiankun
- Luigi
- FrintJS
- PuzzleJS
- Icestark
Sometimes, if we want to display another prepared page, e.g. youtube or an application prepared by us, we can use the html5 iframe element.
The iframe element is used to embed external documents into web pages.
Unfortunately, such a solution is simple and quick, it may not work when we want to display another application on our website, and the external application does not allow it, then we receive the used message.
3. Practical approach to micro frontends architecture. source code, examples
We will create a simple application that will use several popular frameworks. For example, we will use the sample single SPA framework https://single-spa.js.org/
For a simple micro frontend application, I will use the Visual Studio Code development environment(you can always use such as Visual Studio Express or a paid solution from Jetbrains.
Please remember that I will not create all the files for this example, e.g. package.json or other.
Let’s start
I open visual studio code and then a terminal(shortcut ctrl + shift+ `)
Create a new place for a project:
mkdir D:\example cd D:\example code -r .
Installing Regular Depedencies (depending on frameworks we use react, vue)
npm install react react-dom single-spa single-spa-react single-spa-vue vue
Installing Babel Dependencies
npm install @babel/core @babel/plugin-proposal-object-rest-spread @babel/plugin-syntax-dynamic-import
Installing Webpack Dependencies
npm install webpack webpack-cli webpack-dev-server clean-webpack-plugin css-loader html-loader style
Create a folder where we will store projects of selected frameworks
mkdir src mkdir src/vue mkdir src/react
Create webpack config file
code webpack.config.js const path = require('path'); const webpack = require('webpack'); const { CleanWebpackPlugin } = require('clean-webpack-plugin'); const VueLoaderPlugin = require('vue-loader/lib/plugin') module.exports = { mode: 'development', entry: { 'single-spa.config': './single-spa.config.js', }, output: { publicPath: '/dist/', filename: '[name].js', path: path.resolve(__dirname, 'dist'), }, module: { rules: [ { test: /\.css$/, use: ['style-loader', 'css-loader'] }, { test: /\.js$/, exclude: [path.resolve(__dirname, 'node_modules')], loader: 'babel-loader', }, { test: /\.vue$/, loader: 'vue-loader' } ], }, node: { fs: 'empty' }, resolve: { alias: { vue: 'vue/dist/vue.js' }, modules: [path.resolve(__dirname, 'node_modules')], }, plugins: [ new CleanWebpackPlugin(), new VueLoaderPlugin() ], devtool: 'source-map', externals: [], devServer: { historyApiFallback: true } };
Create babel configuration
code .babelrc
{ "presets": [ ["@babel/preset-env", { "targets": { "browsers": ["last 2 versions"] } }], ["@babel/preset-react"] ], "plugins": [ "@babel/plugin-syntax-dynamic-import", "@babel/plugin-proposal-object-rest-spread" ] }
Create single-spa configuration
Code single-spa.config.js
import { registerApplication, start } from 'single-spa' registerApplication( 'vue', () => import('./src/vue/vue.app.js'), () => location.pathname === "/react" ? false : true ); registerApplication( 'react', () => import('./src/react/main.app.js'), () => location.pathname === "/vue" ? false : true ); start();
Create project files into react and vue folders like
cd /src/react
code main.app.js root.component.js
main.app.js
import React from 'react'; import ReactDOM from 'react-dom'; import singleSpaReact from 'single-spa-react'; import Home from './root.component.js'; function getDocument() { return document.getElementById("react") } const reactLifecycles = singleSpaReact({ React, ReactDOM, rootComponent: Home, getDocument, }) export const bootstrap = [ reactLifecycles.bootstrap, ]; export const mount = [ reactLifecycles.mount, ]; export const unmount = [ reactLifecycles.unmount, ];
root.component.js
import React from "react" const App = () => <h1>Hello World from react </h1> export default App
Similarly, we generate a new project for vue
Create Index.html
<html> <head></head> <body> <div id="react"></div> <div id="vue"></div> <script src="/dist/single-spa.config.js"></script> </body> </html>
Run the start script ang go to the browser
npm start
This is the simplest possible example.
In addition to our applications, we can implement appropriate CSS styles to make our application look appropriate.
Another example to create micro frontend application is use single-spa CLI.
https://single-spa.js.org/docs/getting-started-overview
In terminal
npx create-single-spa
The installer will guide you through installing add-ons and dependencies by asking step-by-step questions.
4. Summary
The given entry is only a small fragment introducing you to design an application with the use of a micro frontends.
In conclusion, I want to say that we have access to many great studies on how to build modern web applications with using micro frontend approach. Remember that monolith architecture is not a bad choice.
It all depends on the specifications of the project and the team working on it, you should facilitate and simplify solutions as much as possible.